Create a simple quiz app using Json in Sketchware
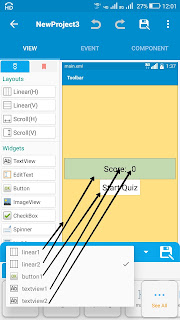
To create a simple quiz app using Json String in Sketchware, follow the steps given below. 1. Create a json array containing all the questions and options. Each object in the array should contain a question with key ' ques ', four options for the question with keys ' a ', ' b ', ' c ' and ' d ', and the correct answer with key ' ans '. [ { "ques" : "The larynx in adults lies in front of hypopharynx opposite the...", "a" : "second to fifth cervical vertebrae", "b" : "fifth to seventh cervical vertebrae", "c" : "third to sixth cervical vertebrae", "d" : "first two cervical vertebrae", "ans" : "c" }, { "ques" : "Which of the following is the largest cartilage of larynx?", "a" : "thyroid cartilage", "b" : "cricoid cartilage", "c" : ...