Display List Map in RecyclerView
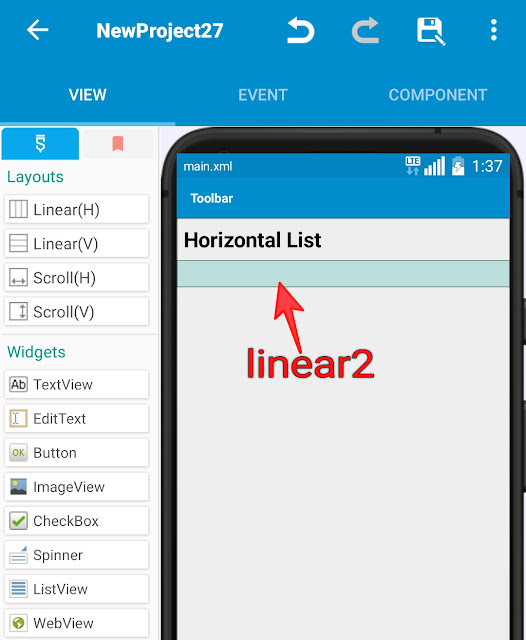
This post describes a sample project which uses RecyclerView to display a List Map containing keys 'text' and 'image_url'. 1. In main.xml add a Linear linear2 for displaying the RecyclerView. 2. Create a CustomView custom_item.xml . In this add a Linear linear1 , an ImageView c_imageview1 , and a TextView c_textview1 . 3. Switch On AppCompat and Design . 4. Create a More block extra . In this block put codes to declare a RecyclerView recyclerView . } androidx.recyclerview.widget.RecyclerView recyclerView; { 5. In onCreate use following codes. // Define recyclerView . recyclerView = new androidx.recyclerview.widget.RecyclerView(this); // Add RecyclerView to linear2 . linear2 .addView(recyclerView); 6. Suppose you have a List Map maplist containing key ' text ' for some text and key ' image_url ' for url of image. 7. Create another More block adapter , and put following codes in it. // This co...