Custom Toast message using CustomView in Sketchware
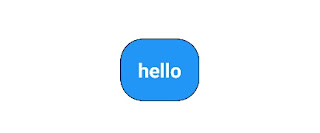
To create a custom Toast message in Sketchware, follow the steps given below. 1. In your Sketchware android project, in VIEW area, create a new CustomView with name custom1. In this CustomView add a LinearH linear1 and a TextView textview1. Customize the textview1 and linear1 as per your requirement. 2. In LOGIC area, add a new More Block with name customToast(String _text) as shown in image below. 3. Next define this customToast Block. In event customToast MoreBlock use add source directly block, and in the block, write codes as described below. First define a new LayoutInflater and use it to create a View from the CustomView. LayoutInflater inflater = getLayoutInflater(); View toastLayout = inflater.inflate(R.layout.custom1, null); Initialize textview1 and linear1 used in CustomView. TextView textview1 = (TextView) toastLayout.findViewById(R.id.textview1); LinearLayout linear1 = (LinearLayout) toastLayout.findViewById(R.id.linear1); Set the text of tex...